In the dynamic world of web development, choosing the right framework is crucial for building scalable and efficient applications. One such framework that has gained prominence in the Node.js ecosystem is Express.js. In this article, we embark on a journey to unveil the basics of Express.js, exploring its origins, core concepts, and practical applications.
The Evolution of Express.js
Historical Context
Express.js emerged as a response to the growing need for a lightweight and unopinionated web framework for Node.js. Its creator, TJ Holowaychuk, envisioned a framework that prioritized simplicity and flexibility, allowing developers to structure their applications as they saw fit.
Node.js and the Rise of Server-Side JavaScript
To understand Express.js, we must first appreciate the role of Node.js. Node.js revolutionized server-side JavaScript, allowing developers to use JavaScript beyond the confines of the browser. With its event-driven, non-blocking I/O model, Node.js became the foundation for building fast and scalable network applications.
TJ Holowaychuk’s Contribution
TJ Holowaychuk, known for his contributions to the Node.js ecosystem, created Express.js in 2010. He aimed to provide developers with a minimal and flexible framework that streamlined the process of building web applications and APIs.
What is Express.js?
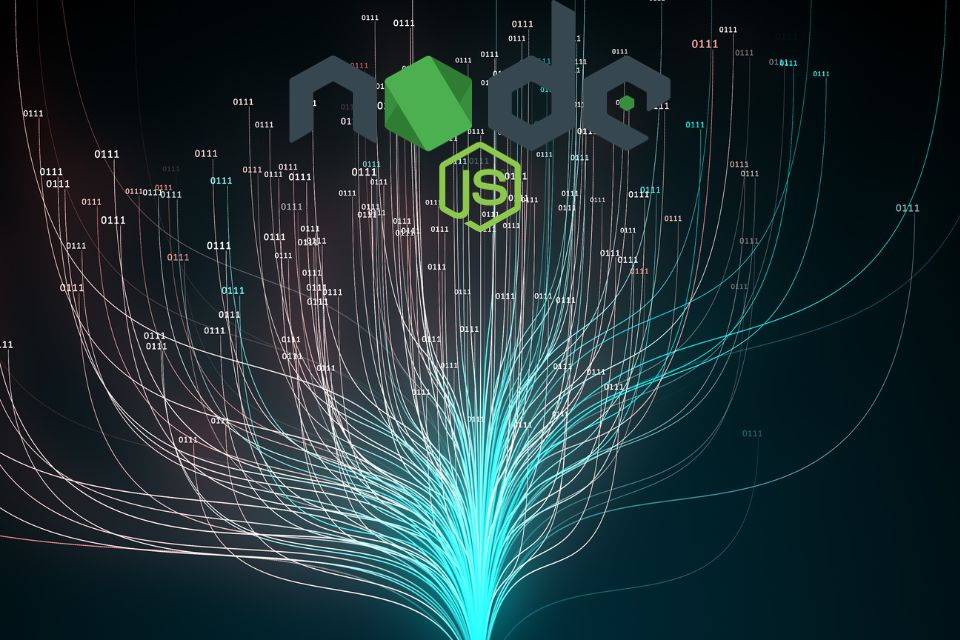
A Node.js web application framework called Express.js makes creating scalable and reliable server-side applications easier. Often referred to as a “micro” framework, Express.js is unopinionated, providing the essentials for web development without imposing strict conventions on the project structure.
Minimalistic Design
Express.js embraces a minimalistic design philosophy, empowering developers to make architectural decisions based on their preferences. It serves as a foundation rather than a complete solution, allowing for modular additions.
Comparison with Other Node.js Frameworks
In the landscape of Node.js frameworks, Express.js stands out for its simplicity and widespread adoption. While frameworks like Koa and Hapi offer unique features and opinions, Express.js remains popular for its ease of use and flexibility.
Core Concepts of Express.js
Middleware
At the heart of Express.js is the concept of middleware. Components with access to the request and response objects and the ability to change them or end the request-response cycle are known as middleware functions. This modular approach enables developers to structure their applications with reusable and composable units.
Routing
Express.js simplifies routing by providing a clean and expressive API for defining endpoints. Developers can specify how the application should respond to different HTTP methods and route parameters. This makes it easy to create RESTful APIs and handle dynamic content.
Request and Response Objects
The request and response objects encapsulate information about the client’s request and provide a response mechanism. Understanding these objects is fundamental to building effective Express.js applications, as they carry essential data throughout the request-response lifecycle.
Setting Up an Express.js Project
To unleash the power of Express.js, developers must first set up a project. Let’s walk through the essential steps.
Installing Express.js
Express.js can be installed using npm, the Node.js package manager. The following command installs Express.js as a dependency for your project:
bashCopy code
npm install express
Initializing a Basic Express.js Project
Once installed, developers can create a basic Express.js project by initializing the application, defining routes, and starting the server. The structure of an Express.js project typically includes files for routes, middleware, and configurations.
Configuration Options
Express.js offers various configuration options to tailor the development environment to specific needs. From setting the port number to configuring middleware, understanding these options is crucial for optimizing the Express.js application.
Routing in Express.js
Routing is a fundamental aspect of web development, and Express.js makes it intuitive and flexible.
Creating Routes
Express.js allows developers to define routes for HTTP methods, such as GET, POST, PUT, and DELETE. Each route corresponds to a specific endpoint, enabling the application to handle various requests.
Parameterized Routes
Parameterized routes in Express.js facilitate the handling of dynamic data. By defining route parameters, developers can create more versatile, reusable routes that respond to different inputs.
Handling Route Parameters and Query Strings
Express.js simplifies the extraction of route parameters and query strings, making accessing and utilizing data from the client’s request easy. This capability is essential for building dynamic and data-driven applications.
Middleware in Action
Middleware is a key concept in Express.js, pivotal in the request-response lifecycle.
Role of Middleware
Middleware functions in Express.js have access to the request and response objects and the ability to modify them. This enables developers to insert custom logic at various points in the processing pipeline, such as authentication, logging, and error handling.
Creating Custom Middleware Functions
Developers can create custom middleware functions tailored to the specific needs of their application. These functions can be added to the middleware stack to execute in sequential order while processing a request.
Implementing Common Middleware
Express.js provides a set of built-in middleware functions that address common requirements. These include middleware for parsing incoming requests, handling cookies, and serving static files. Leveraging these functions enhances the efficiency and security of Express.js applications.
Working with Templates and Views
Express.js seamlessly integrates with template engines, allowing developers to generate dynamic content for views.
Introduction to Template Engines
Template engines, such as Pug (formerly Jade) and EJS, enable the creation of dynamic HTML content. Express.js supports various template engines, providing flexibility in choosing the one that aligns with the developer’s preferences.
Configuring and Using Template Engines
Integrating a template engine with Express.js involves configuring the application to use the engine and defining routes that render dynamic views. This capability is crucial for building web applications with dynamic and data-driven user interfaces.
Integrating Views into Express.js Routes
Express.js routes can render views by combining data with templates. Through this technique, developers may produce interactive, aesthetically pleasing user interfaces that react to data changes and user input.
Interacting with Databases
Building real-world applications often involves interacting with databases to store and retrieve data.
Connecting Express.js Applications to Databases
Express.js supports various databases, including MongoDB, MySQL, and PostgreSQL. Establishing a connection between an Express.js application and a database is fundamental in building data-driven applications.
Performing CRUD Operations
Express.js routes can be used to define endpoints for performing CRUD (Create, Read, Update, Delete) operations on the database. This enables the application to handle user input, store data, and retrieve necessary information.
Best Practices for Database Interactions
Developers should follow best practices to guarantee the effectiveness and security of database interactions. These include using connection pooling, handling errors gracefully, and securing sensitive information such as database credentials.
Error Handling and Debugging
Error handling is critical to building robust applications, and Express.js provides mechanisms to address errors effectively.
Implementing Error Handling Middleware
Express.js allows developers to create custom error-handling middleware to catch and process errors. This middleware can be strategically placed in the middleware stack to ensure comprehensive error coverage.
Debugging Techniques
Debugging is integral to the development process, and Express.js offers tools and techniques to identify and resolve issues. Developers can use debugging modules, logging, and error-tracking services to streamline the debugging process.
Common Pitfalls and Best Practices
Understanding common pitfalls in Express.js development is essential for avoiding potential issues. Best practices, such as validating user input, handling asynchronous code, and implementing secure authentication, contribute to the stability of Express.js applications.
Security Considerations
Securing an Express.js application is paramount to protect against potential vulnerabilities.
Overview of Security Best Practices
Express.js applications should adhere to security best practices to mitigate risks such as Cross-Site Scripting (XSS), Cross-Site Request Forgery (CSRF), and SQL injection. Implementing secure coding practices and staying informed about potential vulnerabilities is crucial.
Preventing Common Vulnerabilities
Express.js provides middleware and configurations to address common security vulnerabilities. Developers should configure security headers, sanitize user input, and use libraries that prevent common attacks to fortify the application against potential threats.
Securing Express.js Applications
Securing an Express.js application requires a holistic approach encompassing code-level security practices and server-level configurations. Regular updates, code reviews, and adherence to security guidelines contribute to the overall security posture of an Express.js application.
Express.js in Real-world Applications
To truly appreciate the power of Express.js, let’s explore some real-world applications that leverage this framework.
Case Study: Myntra
Myntra, a leading e-commerce platform, adopted Express.js to build its scalable and performant backend. The framework’s simplicity and flexibility allowed Myntra to handle many concurrent users, delivering a seamless shopping experience.
Case Study: Accenture
Accenture, a global professional services company, utilized Express.js to develop a robust API gateway. The framework’s middleware architecture and efficient routing mechanisms were crucial in managing and orchestrating diverse microservices.
Lessons Learned from Real-world Projects
Real-world Express.js projects offer valuable insights into the framework’s strengths and challenges. Common lessons include the importance of modular design, effective error handling, and the strategic use of middleware for improved maintainability.
Conclusion
In Node.js web frameworks, Express.js is a beacon of simplicity and flexibility. Unveiling the basics of Express.js has allowed us to explore its evolution, core concepts, and practical applications.
From middleware magic to dynamic routing, Express.js empowers developers to build scalable and efficient web applications. As we conclude this journey into the heart of Express.js, it’s essential to recognize its role in shaping the landscape of server-side JavaScript development.
Express.js invites you to express yourself in web development, regardless of your experience level. It offers up a world of possibilities. Armed with the knowledge of Express.js basics, developers can embark on creating powerful, feature-rich applications that leverage the strengths of this versatile Node.js framework.